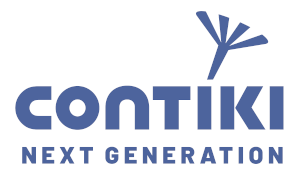 |
Contiki-NG
|
26#ifndef _TUSB_CONFIG_H_
27#define _TUSB_CONFIG_H_
39 #error CFG_TUSB_MCU must be defined
43#ifndef BOARD_DEVICE_RHPORT_NUM
44 #define BOARD_DEVICE_RHPORT_NUM 0
49#ifndef BOARD_DEVICE_RHPORT_SPEED
50 #if (CFG_TUSB_MCU == OPT_MCU_LPC18XX || CFG_TUSB_MCU == OPT_MCU_LPC43XX || CFG_TUSB_MCU == OPT_MCU_MIMXRT10XX || \
51 CFG_TUSB_MCU == OPT_MCU_NUC505 || CFG_TUSB_MCU == OPT_MCU_CXD56)
52 #define BOARD_DEVICE_RHPORT_SPEED OPT_MODE_HIGH_SPEED
54 #define BOARD_DEVICE_RHPORT_SPEED OPT_MODE_FULL_SPEED
59#if BOARD_DEVICE_RHPORT_NUM == 0
60 #define CFG_TUSB_RHPORT0_MODE (OPT_MODE_DEVICE | BOARD_DEVICE_RHPORT_SPEED)
61#elif BOARD_DEVICE_RHPORT_NUM == 1
62 #define CFG_TUSB_RHPORT1_MODE (OPT_MODE_DEVICE | BOARD_DEVICE_RHPORT_SPEED)
64 #error "Incorrect RHPort configuration"
69#define CFG_TUSB_OS OPT_OS_NONE
82#ifndef CFG_TUSB_MEM_SECTION
83#define CFG_TUSB_MEM_SECTION
86#ifndef CFG_TUSB_MEM_ALIGN
87#define CFG_TUSB_MEM_ALIGN __attribute__ ((aligned(4)))
94#ifndef CFG_TUD_ENDPOINT0_SIZE
95#define CFG_TUD_ENDPOINT0_SIZE 64
102#define CFG_TUD_MIDI 0
103#define CFG_TUD_VENDOR 0
106#define CFG_TUD_CDC_RX_BUFSIZE (TUD_OPT_HIGH_SPEED ? 512 : 64)
107#define CFG_TUD_CDC_TX_BUFSIZE (TUD_OPT_HIGH_SPEED ? 512 : 64)
110#define CFG_TUD_CDC_EP_BUFSIZE (TUD_OPT_HIGH_SPEED ? 512 : 64)
113#define CFG_TUD_MSC_EP_BUFSIZE 512