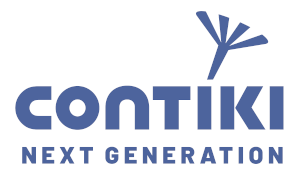 |
Contiki-NG
|
34#ifndef NRFX_CONFIG_NRF5340_APPLICATION_H__
35#define NRFX_CONFIG_NRF5340_APPLICATION_H__
37#ifndef NRFX_CONFIG_H__
38#error "This file should not be included directly. Include nrfx_config.h instead."
41#if defined(NRF_TRUSTZONE_NONSECURE)
42#define NRF_PERIPH(P) P##_NS
44#define NRF_PERIPH(P) P##_S
54#define NRF_CLOCK NRF_PERIPH(NRF_CLOCK)
55#define NRF_COMP NRF_PERIPH(NRF_COMP)
56#define NRF_DCNF NRF_PERIPH(NRF_DCNF)
57#define NRF_DPPIC NRF_PERIPH(NRF_DPPIC)
58#define NRF_EGU0 NRF_PERIPH(NRF_EGU0)
59#define NRF_EGU1 NRF_PERIPH(NRF_EGU1)
60#define NRF_EGU2 NRF_PERIPH(NRF_EGU2)
61#define NRF_EGU3 NRF_PERIPH(NRF_EGU3)
62#define NRF_EGU4 NRF_PERIPH(NRF_EGU4)
63#define NRF_EGU5 NRF_PERIPH(NRF_EGU5)
64#define NRF_FPU NRF_PERIPH(NRF_FPU)
65#define NRF_I2S0 NRF_PERIPH(NRF_I2S0)
66#define NRF_IPC NRF_PERIPH(NRF_IPC)
67#define NRF_KMU NRF_PERIPH(NRF_KMU)
68#define NRF_LPCOMP NRF_PERIPH(NRF_LPCOMP)
69#define NRF_MUTEX NRF_PERIPH(NRF_MUTEX)
70#define NRF_NFCT NRF_PERIPH(NRF_NFCT)
71#define NRF_NVMC NRF_PERIPH(NRF_NVMC)
72#define NRF_OSCILLATORS NRF_PERIPH(NRF_OSCILLATORS)
73#define NRF_P0 NRF_PERIPH(NRF_P0)
74#define NRF_P1 NRF_PERIPH(NRF_P1)
75#define NRF_PDM0 NRF_PERIPH(NRF_PDM0)
76#define NRF_POWER NRF_PERIPH(NRF_POWER)
77#define NRF_PWM0 NRF_PERIPH(NRF_PWM0)
78#define NRF_PWM1 NRF_PERIPH(NRF_PWM1)
79#define NRF_PWM2 NRF_PERIPH(NRF_PWM2)
80#define NRF_PWM3 NRF_PERIPH(NRF_PWM3)
81#define NRF_QDEC0 NRF_PERIPH(NRF_QDEC0)
82#define NRF_QDEC1 NRF_PERIPH(NRF_QDEC1)
83#define NRF_QSPI NRF_PERIPH(NRF_QSPI)
84#define NRF_REGULATORS NRF_PERIPH(NRF_REGULATORS)
85#define NRF_RESET NRF_PERIPH(NRF_RESET)
86#define NRF_RTC0 NRF_PERIPH(NRF_RTC0)
87#define NRF_RTC1 NRF_PERIPH(NRF_RTC1)
88#define NRF_SAADC NRF_PERIPH(NRF_SAADC)
89#define NRF_SPIM0 NRF_PERIPH(NRF_SPIM0)
90#define NRF_SPIM1 NRF_PERIPH(NRF_SPIM1)
91#define NRF_SPIM2 NRF_PERIPH(NRF_SPIM2)
92#define NRF_SPIM3 NRF_PERIPH(NRF_SPIM3)
93#define NRF_SPIM4 NRF_PERIPH(NRF_SPIM4)
94#define NRF_SPIS0 NRF_PERIPH(NRF_SPIS0)
95#define NRF_SPIS1 NRF_PERIPH(NRF_SPIS1)
96#define NRF_SPIS2 NRF_PERIPH(NRF_SPIS2)
97#define NRF_SPIS3 NRF_PERIPH(NRF_SPIS3)
98#define NRF_TIMER0 NRF_PERIPH(NRF_TIMER0)
99#define NRF_TIMER1 NRF_PERIPH(NRF_TIMER1)
100#define NRF_TIMER2 NRF_PERIPH(NRF_TIMER2)
101#define NRF_TWIM0 NRF_PERIPH(NRF_TWIM0)
102#define NRF_TWIM1 NRF_PERIPH(NRF_TWIM1)
103#define NRF_TWIM2 NRF_PERIPH(NRF_TWIM2)
104#define NRF_TWIM3 NRF_PERIPH(NRF_TWIM3)
105#define NRF_TWIS0 NRF_PERIPH(NRF_TWIS0)
106#define NRF_TWIS1 NRF_PERIPH(NRF_TWIS1)
107#define NRF_TWIS2 NRF_PERIPH(NRF_TWIS2)
108#define NRF_TWIS3 NRF_PERIPH(NRF_TWIS3)
109#define NRF_UARTE0 NRF_PERIPH(NRF_UARTE0)
110#define NRF_UARTE1 NRF_PERIPH(NRF_UARTE1)
111#define NRF_UARTE2 NRF_PERIPH(NRF_UARTE2)
112#define NRF_UARTE3 NRF_PERIPH(NRF_UARTE3)
113#define NRF_USBD NRF_PERIPH(NRF_USBD)
114#define NRF_USBREGULATOR NRF_PERIPH(NRF_USBREGULATOR)
115#define NRF_VMC NRF_PERIPH(NRF_VMC)
116#define NRF_WDT0 NRF_PERIPH(NRF_WDT0)
117#define NRF_WDT1 NRF_PERIPH(NRF_WDT1)
124#if defined(NRF_TRUSTZONE_NONSECURE)
125#define NRF_GPIOTE1 NRF_GPIOTE1_NS
127#define NRF_CACHE NRF_CACHE_S
128#define NRF_CACHEINFO NRF_CACHEINFO_S
129#define NRF_CACHEDATA NRF_CACHEDATA_S
130#define NRF_CRYPTOCELL NRF_CRYPTOCELL_S
131#define NRF_CTI NRF_CTI_S
132#define NRF_FICR NRF_FICR_S
133#define NRF_GPIOTE0 NRF_GPIOTE0_S
134#define NRF_SPU NRF_SPU_S
135#define NRF_TAD NRF_TAD_S
136#define NRF_UICR NRF_UICR_S
140#if defined(NRF_TRUSTZONE_NONSECURE)
141#define NRF_GPIOTE NRF_GPIOTE1
142#define GPIOTE_IRQHandler GPIOTE1_IRQHandler
144#define NRF_GPIOTE NRF_GPIOTE0
145#define GPIOTE_IRQHandler GPIOTE0_IRQHandler
149#define NRF_QDEC NRF_QDEC0
150#define QDEC_IRQHandler QDEC0_IRQHandler
158#ifndef NRFX_CLOCK_ENABLED
159#define NRFX_CLOCK_ENABLED 0
168#ifndef NRFX_CLOCK_CONFIG_LF_SRC
169#define NRFX_CLOCK_CONFIG_LF_SRC 2
174#ifndef NRFX_CLOCK_CONFIG_LF_CAL_ENABLED
175#define NRFX_CLOCK_CONFIG_LF_CAL_ENABLED 0
185#ifndef NRFX_CLOCK_CONFIG_LFXO_TWO_STAGE_ENABLED
186#define NRFX_CLOCK_CONFIG_LFXO_TWO_STAGE_ENABLED 0
194#ifndef NRFX_CLOCK_CONFIG_HFCLK192M_SRC
195#define NRFX_CLOCK_CONFIG_HFCLK192M_SRC 1
209#ifndef NRFX_CLOCK_DEFAULT_CONFIG_IRQ_PRIORITY
210#define NRFX_CLOCK_DEFAULT_CONFIG_IRQ_PRIORITY 7
215#ifndef NRFX_CLOCK_CONFIG_LOG_ENABLED
216#define NRFX_CLOCK_CONFIG_LOG_ENABLED 0
226#ifndef NRFX_CLOCK_CONFIG_LOG_LEVEL
227#define NRFX_CLOCK_CONFIG_LOG_LEVEL 3
242#ifndef NRFX_CLOCK_CONFIG_INFO_COLOR
243#define NRFX_CLOCK_CONFIG_INFO_COLOR 0
258#ifndef NRFX_CLOCK_CONFIG_DEBUG_COLOR
259#define NRFX_CLOCK_CONFIG_DEBUG_COLOR 0
268#ifndef NRFX_COMP_ENABLED
269#define NRFX_COMP_ENABLED 0
283#ifndef NRFX_COMP_DEFAULT_CONFIG_IRQ_PRIORITY
284#define NRFX_COMP_DEFAULT_CONFIG_IRQ_PRIORITY 7
289#ifndef NRFX_COMP_CONFIG_LOG_ENABLED
290#define NRFX_COMP_CONFIG_LOG_ENABLED 0
300#ifndef NRFX_COMP_CONFIG_LOG_LEVEL
301#define NRFX_COMP_CONFIG_LOG_LEVEL 3
316#ifndef NRFX_COMP_CONFIG_INFO_COLOR
317#define NRFX_COMP_CONFIG_INFO_COLOR 0
332#ifndef NRFX_COMP_CONFIG_DEBUG_COLOR
333#define NRFX_COMP_CONFIG_DEBUG_COLOR 0
342#ifndef NRFX_DPPI_ENABLED
343#define NRFX_DPPI_ENABLED 0
347#ifndef NRFX_DPPI_CONFIG_LOG_ENABLED
348#define NRFX_DPPI_CONFIG_LOG_ENABLED 0
358#ifndef NRFX_DPPI_CONFIG_LOG_LEVEL
359#define NRFX_DPPI_CONFIG_LOG_LEVEL 3
374#ifndef NRFX_DPPI_CONFIG_INFO_COLOR
375#define NRFX_DPPI_CONFIG_INFO_COLOR 0
390#ifndef NRFX_DPPI_CONFIG_DEBUG_COLOR
391#define NRFX_DPPI_CONFIG_DEBUG_COLOR 0
400#ifndef NRFX_EGU_ENABLED
401#define NRFX_EGU_ENABLED 0
406#ifndef NRFX_EGU0_ENABLED
407#define NRFX_EGU0_ENABLED 0
412#ifndef NRFX_EGU1_ENABLED
413#define NRFX_EGU1_ENABLED 0
418#ifndef NRFX_EGU2_ENABLED
419#define NRFX_EGU2_ENABLED 0
424#ifndef NRFX_EGU3_ENABLED
425#define NRFX_EGU3_ENABLED 0
430#ifndef NRFX_EGU4_ENABLED
431#define NRFX_EGU4_ENABLED 0
436#ifndef NRFX_EGU5_ENABLED
437#define NRFX_EGU5_ENABLED 0
451#ifndef NRFX_EGU_DEFAULT_CONFIG_IRQ_PRIORITY
452#define NRFX_EGU_DEFAULT_CONFIG_IRQ_PRIORITY 7
459#ifndef NRFX_GPIOTE_ENABLED
460#define NRFX_GPIOTE_ENABLED 0
463#ifndef NRFX_GPIOTE_CONFIG_NUM_OF_LOW_POWER_EVENTS
464#define NRFX_GPIOTE_CONFIG_NUM_OF_LOW_POWER_EVENTS 1
478#ifndef NRFX_GPIOTE_DEFAULT_CONFIG_IRQ_PRIORITY
479#define NRFX_GPIOTE_DEFAULT_CONFIG_IRQ_PRIORITY 7
484#ifndef NRFX_GPIOTE_CONFIG_LOG_ENABLED
485#define NRFX_GPIOTE_CONFIG_LOG_ENABLED 0
495#ifndef NRFX_GPIOTE_CONFIG_LOG_LEVEL
496#define NRFX_GPIOTE_CONFIG_LOG_LEVEL 3
511#ifndef NRFX_GPIOTE_CONFIG_INFO_COLOR
512#define NRFX_GPIOTE_CONFIG_INFO_COLOR 0
527#ifndef NRFX_GPIOTE_CONFIG_DEBUG_COLOR
528#define NRFX_GPIOTE_CONFIG_DEBUG_COLOR 0
537#ifndef NRFX_I2S_ENABLED
538#define NRFX_I2S_ENABLED 0
552#ifndef NRFX_I2S_DEFAULT_CONFIG_IRQ_PRIORITY
553#define NRFX_I2S_DEFAULT_CONFIG_IRQ_PRIORITY 7
558#ifndef NRFX_I2S_CONFIG_LOG_ENABLED
559#define NRFX_I2S_CONFIG_LOG_ENABLED 0
569#ifndef NRFX_I2S_CONFIG_LOG_LEVEL
570#define NRFX_I2S_CONFIG_LOG_LEVEL 3
585#ifndef NRFX_I2S_CONFIG_INFO_COLOR
586#define NRFX_I2S_CONFIG_INFO_COLOR 0
601#ifndef NRFX_I2S_CONFIG_DEBUG_COLOR
602#define NRFX_I2S_CONFIG_DEBUG_COLOR 0
611#ifndef NRFX_IPC_ENABLED
612#define NRFX_IPC_ENABLED 0
619#ifndef NRFX_LPCOMP_ENABLED
620#define NRFX_LPCOMP_ENABLED 0
634#ifndef NRFX_LPCOMP_DEFAULT_CONFIG_IRQ_PRIORITY
635#define NRFX_LPCOMP_DEFAULT_CONFIG_IRQ_PRIORITY 7
640#ifndef NRFX_LPCOMP_CONFIG_LOG_ENABLED
641#define NRFX_LPCOMP_CONFIG_LOG_ENABLED 0
651#ifndef NRFX_LPCOMP_CONFIG_LOG_LEVEL
652#define NRFX_LPCOMP_CONFIG_LOG_LEVEL 3
667#ifndef NRFX_LPCOMP_CONFIG_INFO_COLOR
668#define NRFX_LPCOMP_CONFIG_INFO_COLOR 0
683#ifndef NRFX_LPCOMP_CONFIG_DEBUG_COLOR
684#define NRFX_LPCOMP_CONFIG_DEBUG_COLOR 0
693#ifndef NRFX_NFCT_ENABLED
694#define NRFX_NFCT_ENABLED 0
707#ifndef NRFX_NFCT_DEFAULT_CONFIG_IRQ_PRIORITY
708#define NRFX_NFCT_DEFAULT_CONFIG_IRQ_PRIORITY 7
717#ifndef NRFX_NFCT_CONFIG_TIMER_INSTANCE_ID
718#define NRFX_NFCT_CONFIG_TIMER_INSTANCE_ID 2
723#ifndef NRFX_NFCT_CONFIG_LOG_ENABLED
724#define NRFX_NFCT_CONFIG_LOG_ENABLED 0
734#ifndef NRFX_NFCT_CONFIG_LOG_LEVEL
735#define NRFX_NFCT_CONFIG_LOG_LEVEL 3
750#ifndef NRFX_NFCT_CONFIG_INFO_COLOR
751#define NRFX_NFCT_CONFIG_INFO_COLOR 0
766#ifndef NRFX_NFCT_CONFIG_DEBUG_COLOR
767#define NRFX_NFCT_CONFIG_DEBUG_COLOR 0
776#ifndef NRFX_NVMC_ENABLED
777#define NRFX_NVMC_ENABLED 0
784#ifndef NRFX_PDM_ENABLED
785#define NRFX_PDM_ENABLED 0
799#ifndef NRFX_PDM_DEFAULT_CONFIG_IRQ_PRIORITY
800#define NRFX_PDM_DEFAULT_CONFIG_IRQ_PRIORITY 7
805#ifndef NRFX_PDM_CONFIG_LOG_ENABLED
806#define NRFX_PDM_CONFIG_LOG_ENABLED 0
816#ifndef NRFX_PDM_CONFIG_LOG_LEVEL
817#define NRFX_PDM_CONFIG_LOG_LEVEL 3
832#ifndef NRFX_PDM_CONFIG_INFO_COLOR
833#define NRFX_PDM_CONFIG_INFO_COLOR 0
848#ifndef NRFX_PDM_CONFIG_DEBUG_COLOR
849#define NRFX_PDM_CONFIG_DEBUG_COLOR 0
858#ifndef NRFX_POWER_ENABLED
859#define NRFX_POWER_ENABLED 0
872#ifndef NRFX_POWER_DEFAULT_CONFIG_IRQ_PRIORITY
873#define NRFX_POWER_DEFAULT_CONFIG_IRQ_PRIORITY 7
880#ifndef NRFX_PRS_ENABLED
881#define NRFX_PRS_ENABLED 0
886#ifndef NRFX_PRS_BOX_0_ENABLED
887#define NRFX_PRS_BOX_0_ENABLED 0
893#ifndef NRFX_PRS_BOX_1_ENABLED
894#define NRFX_PRS_BOX_1_ENABLED 0
900#ifndef NRFX_PRS_BOX_2_ENABLED
901#define NRFX_PRS_BOX_2_ENABLED 0
907#ifndef NRFX_PRS_BOX_3_ENABLED
908#define NRFX_PRS_BOX_3_ENABLED 0
914#ifndef NRFX_PRS_BOX_4_ENABLED
915#define NRFX_PRS_BOX_4_ENABLED 0
921#ifndef NRFX_PRS_CONFIG_LOG_ENABLED
922#define NRFX_PRS_CONFIG_LOG_ENABLED 0
932#ifndef NRFX_PRS_CONFIG_LOG_LEVEL
933#define NRFX_PRS_CONFIG_LOG_LEVEL 3
948#ifndef NRFX_PRS_CONFIG_INFO_COLOR
949#define NRFX_PRS_CONFIG_INFO_COLOR 0
964#ifndef NRFX_PRS_CONFIG_DEBUG_COLOR
965#define NRFX_PRS_CONFIG_DEBUG_COLOR 0
974#ifndef NRFX_PWM_ENABLED
975#define NRFX_PWM_ENABLED 0
980#ifndef NRFX_PWM0_ENABLED
981#define NRFX_PWM0_ENABLED 0
987#ifndef NRFX_PWM1_ENABLED
988#define NRFX_PWM1_ENABLED 0
994#ifndef NRFX_PWM2_ENABLED
995#define NRFX_PWM2_ENABLED 0
1001#ifndef NRFX_PWM3_ENABLED
1002#define NRFX_PWM3_ENABLED 0
1016#ifndef NRFX_PWM_DEFAULT_CONFIG_IRQ_PRIORITY
1017#define NRFX_PWM_DEFAULT_CONFIG_IRQ_PRIORITY 7
1022#ifndef NRFX_PWM_CONFIG_LOG_ENABLED
1023#define NRFX_PWM_CONFIG_LOG_ENABLED 0
1033#ifndef NRFX_PWM_CONFIG_LOG_LEVEL
1034#define NRFX_PWM_CONFIG_LOG_LEVEL 3
1049#ifndef NRFX_PWM_CONFIG_INFO_COLOR
1050#define NRFX_PWM_CONFIG_INFO_COLOR 0
1065#ifndef NRFX_PWM_CONFIG_DEBUG_COLOR
1066#define NRFX_PWM_CONFIG_DEBUG_COLOR 0
1075#ifndef NRFX_QDEC_ENABLED
1076#define NRFX_QDEC_ENABLED 0
1090#ifndef NRFX_QDEC_DEFAULT_CONFIG_IRQ_PRIORITY
1091#define NRFX_QDEC_DEFAULT_CONFIG_IRQ_PRIORITY 7
1096#ifndef NRFX_QDEC_CONFIG_LOG_ENABLED
1097#define NRFX_QDEC_CONFIG_LOG_ENABLED 0
1107#ifndef NRFX_QDEC_CONFIG_LOG_LEVEL
1108#define NRFX_QDEC_CONFIG_LOG_LEVEL 3
1123#ifndef NRFX_QDEC_CONFIG_INFO_COLOR
1124#define NRFX_QDEC_CONFIG_INFO_COLOR 0
1139#ifndef NRFX_QDEC_CONFIG_DEBUG_COLOR
1140#define NRFX_QDEC_CONFIG_DEBUG_COLOR 0
1149#ifndef NRFX_QSPI_ENABLED
1150#define NRFX_QSPI_ENABLED 0
1164#ifndef NRFX_QSPI_DEFAULT_CONFIG_IRQ_PRIORITY
1165#define NRFX_QSPI_DEFAULT_CONFIG_IRQ_PRIORITY 7
1172#ifndef NRFX_RTC_ENABLED
1173#define NRFX_RTC_ENABLED 0
1178#ifndef NRFX_RTC0_ENABLED
1179#define NRFX_RTC0_ENABLED 0
1185#ifndef NRFX_RTC1_ENABLED
1186#define NRFX_RTC1_ENABLED 0
1200#ifndef NRFX_RTC_DEFAULT_CONFIG_IRQ_PRIORITY
1201#define NRFX_RTC_DEFAULT_CONFIG_IRQ_PRIORITY 7
1206#ifndef NRFX_RTC_CONFIG_LOG_ENABLED
1207#define NRFX_RTC_CONFIG_LOG_ENABLED 0
1217#ifndef NRFX_RTC_CONFIG_LOG_LEVEL
1218#define NRFX_RTC_CONFIG_LOG_LEVEL 3
1233#ifndef NRFX_RTC_CONFIG_INFO_COLOR
1234#define NRFX_RTC_CONFIG_INFO_COLOR 0
1249#ifndef NRFX_RTC_CONFIG_DEBUG_COLOR
1250#define NRFX_RTC_CONFIG_DEBUG_COLOR 0
1259#ifndef NRFX_SAADC_ENABLED
1260#define NRFX_SAADC_ENABLED 0
1274#ifndef NRFX_SAADC_DEFAULT_CONFIG_IRQ_PRIORITY
1275#define NRFX_SAADC_DEFAULT_CONFIG_IRQ_PRIORITY 7
1280#ifndef NRFX_SAADC_CONFIG_LOG_ENABLED
1281#define NRFX_SAADC_CONFIG_LOG_ENABLED 0
1291#ifndef NRFX_SAADC_CONFIG_LOG_LEVEL
1292#define NRFX_SAADC_CONFIG_LOG_LEVEL 3
1307#ifndef NRFX_SAADC_CONFIG_INFO_COLOR
1308#define NRFX_SAADC_CONFIG_INFO_COLOR 0
1323#ifndef NRFX_SAADC_CONFIG_DEBUG_COLOR
1324#define NRFX_SAADC_CONFIG_DEBUG_COLOR 0
1333#ifndef NRFX_SPIM_ENABLED
1334#define NRFX_SPIM_ENABLED 0
1339#ifndef NRFX_SPIM0_ENABLED
1340#define NRFX_SPIM0_ENABLED 0
1346#ifndef NRFX_SPIM1_ENABLED
1347#define NRFX_SPIM1_ENABLED 0
1353#ifndef NRFX_SPIM2_ENABLED
1354#define NRFX_SPIM2_ENABLED 0
1360#ifndef NRFX_SPIM3_ENABLED
1361#define NRFX_SPIM3_ENABLED 0
1367#ifndef NRFX_SPIM4_ENABLED
1368#define NRFX_SPIM4_ENABLED 0
1374#ifndef NRFX_SPIM_EXTENDED_ENABLED
1375#define NRFX_SPIM_EXTENDED_ENABLED 0
1389#ifndef NRFX_SPIM_DEFAULT_CONFIG_IRQ_PRIORITY
1390#define NRFX_SPIM_DEFAULT_CONFIG_IRQ_PRIORITY 7
1395#ifndef NRFX_SPIM_CONFIG_LOG_ENABLED
1396#define NRFX_SPIM_CONFIG_LOG_ENABLED 0
1406#ifndef NRFX_SPIM_CONFIG_LOG_LEVEL
1407#define NRFX_SPIM_CONFIG_LOG_LEVEL 3
1422#ifndef NRFX_SPIM_CONFIG_INFO_COLOR
1423#define NRFX_SPIM_CONFIG_INFO_COLOR 0
1438#ifndef NRFX_SPIM_CONFIG_DEBUG_COLOR
1439#define NRFX_SPIM_CONFIG_DEBUG_COLOR 0
1448#ifndef NRFX_SPIS_ENABLED
1449#define NRFX_SPIS_ENABLED 0
1454#ifndef NRFX_SPIS0_ENABLED
1455#define NRFX_SPIS0_ENABLED 0
1461#ifndef NRFX_SPIS1_ENABLED
1462#define NRFX_SPIS1_ENABLED 0
1468#ifndef NRFX_SPIS2_ENABLED
1469#define NRFX_SPIS2_ENABLED 0
1475#ifndef NRFX_SPIS3_ENABLED
1476#define NRFX_SPIS3_ENABLED 0
1490#ifndef NRFX_SPIS_DEFAULT_CONFIG_IRQ_PRIORITY
1491#define NRFX_SPIS_DEFAULT_CONFIG_IRQ_PRIORITY 7
1496#ifndef NRFX_SPIS_CONFIG_LOG_ENABLED
1497#define NRFX_SPIS_CONFIG_LOG_ENABLED 0
1507#ifndef NRFX_SPIS_CONFIG_LOG_LEVEL
1508#define NRFX_SPIS_CONFIG_LOG_LEVEL 3
1523#ifndef NRFX_SPIS_CONFIG_INFO_COLOR
1524#define NRFX_SPIS_CONFIG_INFO_COLOR 0
1539#ifndef NRFX_SPIS_CONFIG_DEBUG_COLOR
1540#define NRFX_SPIS_CONFIG_DEBUG_COLOR 0
1550#ifndef NRFX_SYSTICK_ENABLED
1551#define NRFX_SYSTICK_ENABLED 0
1556#ifndef NRFX_TIMER_ENABLED
1557#define NRFX_TIMER_ENABLED 0
1562#ifndef NRFX_TIMER0_ENABLED
1563#define NRFX_TIMER0_ENABLED 0
1569#ifndef NRFX_TIMER1_ENABLED
1570#define NRFX_TIMER1_ENABLED 0
1576#ifndef NRFX_TIMER2_ENABLED
1577#define NRFX_TIMER2_ENABLED 0
1591#ifndef NRFX_TIMER_DEFAULT_CONFIG_IRQ_PRIORITY
1592#define NRFX_TIMER_DEFAULT_CONFIG_IRQ_PRIORITY 7
1597#ifndef NRFX_TIMER_CONFIG_LOG_ENABLED
1598#define NRFX_TIMER_CONFIG_LOG_ENABLED 0
1608#ifndef NRFX_TIMER_CONFIG_LOG_LEVEL
1609#define NRFX_TIMER_CONFIG_LOG_LEVEL 3
1624#ifndef NRFX_TIMER_CONFIG_INFO_COLOR
1625#define NRFX_TIMER_CONFIG_INFO_COLOR 0
1640#ifndef NRFX_TIMER_CONFIG_DEBUG_COLOR
1641#define NRFX_TIMER_CONFIG_DEBUG_COLOR 0
1650#ifndef NRFX_TWIM_ENABLED
1651#define NRFX_TWIM_ENABLED 0
1656#ifndef NRFX_TWIM0_ENABLED
1657#define NRFX_TWIM0_ENABLED 0
1663#ifndef NRFX_TWIM1_ENABLED
1664#define NRFX_TWIM1_ENABLED 0
1670#ifndef NRFX_TWIM2_ENABLED
1671#define NRFX_TWIM2_ENABLED 0
1677#ifndef NRFX_TWIM3_ENABLED
1678#define NRFX_TWIM3_ENABLED 0
1692#ifndef NRFX_TWIM_DEFAULT_CONFIG_IRQ_PRIORITY
1693#define NRFX_TWIM_DEFAULT_CONFIG_IRQ_PRIORITY 7
1698#ifndef NRFX_TWIM_CONFIG_LOG_ENABLED
1699#define NRFX_TWIM_CONFIG_LOG_ENABLED 0
1709#ifndef NRFX_TWIM_CONFIG_LOG_LEVEL
1710#define NRFX_TWIM_CONFIG_LOG_LEVEL 3
1725#ifndef NRFX_TWIM_CONFIG_INFO_COLOR
1726#define NRFX_TWIM_CONFIG_INFO_COLOR 0
1741#ifndef NRFX_TWIM_CONFIG_DEBUG_COLOR
1742#define NRFX_TWIM_CONFIG_DEBUG_COLOR 0
1751#ifndef NRFX_TWIS_ENABLED
1752#define NRFX_TWIS_ENABLED 0
1757#ifndef NRFX_TWIS0_ENABLED
1758#define NRFX_TWIS0_ENABLED 0
1763#ifndef NRFX_TWIS1_ENABLED
1764#define NRFX_TWIS1_ENABLED 0
1769#ifndef NRFX_TWIS2_ENABLED
1770#define NRFX_TWIS2_ENABLED 0
1775#ifndef NRFX_TWIS3_ENABLED
1776#define NRFX_TWIS3_ENABLED 0
1783#ifndef NRFX_TWIS_ASSUME_INIT_AFTER_RESET_ONLY
1784#define NRFX_TWIS_ASSUME_INIT_AFTER_RESET_ONLY 0
1791#ifndef NRFX_TWIS_NO_SYNC_MODE
1792#define NRFX_TWIS_NO_SYNC_MODE 0
1806#ifndef NRFX_TWIS_DEFAULT_CONFIG_IRQ_PRIORITY
1807#define NRFX_TWIS_DEFAULT_CONFIG_IRQ_PRIORITY 7
1812#ifndef NRFX_TWIS_CONFIG_LOG_ENABLED
1813#define NRFX_TWIS_CONFIG_LOG_ENABLED 0
1823#ifndef NRFX_TWIS_CONFIG_LOG_LEVEL
1824#define NRFX_TWIS_CONFIG_LOG_LEVEL 3
1839#ifndef NRFX_TWIS_CONFIG_INFO_COLOR
1840#define NRFX_TWIS_CONFIG_INFO_COLOR 0
1855#ifndef NRFX_TWIS_CONFIG_DEBUG_COLOR
1856#define NRFX_TWIS_CONFIG_DEBUG_COLOR 0
1865#ifndef NRFX_UARTE_ENABLED
1866#define NRFX_UARTE_ENABLED 0
1869#ifndef NRFX_UARTE0_ENABLED
1870#define NRFX_UARTE0_ENABLED 0
1874#ifndef NRFX_UARTE1_ENABLED
1875#define NRFX_UARTE1_ENABLED 0
1879#ifndef NRFX_UARTE2_ENABLED
1880#define NRFX_UARTE2_ENABLED 0
1884#ifndef NRFX_UARTE3_ENABLED
1885#define NRFX_UARTE3_ENABLED 0
1899#ifndef NRFX_UARTE_DEFAULT_CONFIG_IRQ_PRIORITY
1900#define NRFX_UARTE_DEFAULT_CONFIG_IRQ_PRIORITY 7
1905#ifndef NRFX_UARTE_CONFIG_LOG_ENABLED
1906#define NRFX_UARTE_CONFIG_LOG_ENABLED 0
1916#ifndef NRFX_UARTE_CONFIG_LOG_LEVEL
1917#define NRFX_UARTE_CONFIG_LOG_LEVEL 3
1932#ifndef NRFX_UARTE_CONFIG_INFO_COLOR
1933#define NRFX_UARTE_CONFIG_INFO_COLOR 0
1948#ifndef NRFX_UARTE_CONFIG_DEBUG_COLOR
1949#define NRFX_UARTE_CONFIG_DEBUG_COLOR 0
1958#ifndef NRFX_USBD_ENABLED
1959#define NRFX_USBD_ENABLED 0
1972#ifndef NRFX_USBD_DEFAULT_CONFIG_IRQ_PRIORITY
1973#define NRFX_USBD_DEFAULT_CONFIG_IRQ_PRIORITY 7
1984#ifndef NRFX_USBD_CONFIG_DMASCHEDULER_ISO_BOOST
1985#define NRFX_USBD_CONFIG_DMASCHEDULER_ISO_BOOST 1
1994#ifndef NRFX_USBD_CONFIG_ISO_IN_ZLP
1995#define NRFX_USBD_CONFIG_ISO_IN_ZLP 0
2000#ifndef NRFX_USBD_CONFIG_LOG_ENABLED
2001#define NRFX_USBD_CONFIG_LOG_ENABLED 0
2011#ifndef NRFX_USBD_CONFIG_LOG_LEVEL
2012#define NRFX_USBD_CONFIG_LOG_LEVEL 3
2027#ifndef NRFX_USBD_CONFIG_INFO_COLOR
2028#define NRFX_USBD_CONFIG_INFO_COLOR 0
2043#ifndef NRFX_USBD_CONFIG_DEBUG_COLOR
2044#define NRFX_USBD_CONFIG_DEBUG_COLOR 0
2053#ifndef NRFX_USBREG_ENABLED
2054#define NRFX_USBREG_ENABLED 0
2067#ifndef NRFX_USBREG_DEFAULT_CONFIG_IRQ_PRIORITY
2068#define NRFX_USBREG_DEFAULT_CONFIG_IRQ_PRIORITY 7
2077#ifndef NRFX_WDT_ENABLED
2078#define NRFX_WDT_ENABLED 0
2083#ifndef NRFX_WDT0_ENABLED
2084#define NRFX_WDT0_ENABLED 0
2090#ifndef NRFX_WDT1_ENABLED
2091#define NRFX_WDT1_ENABLED 0
2099#ifndef NRFX_WDT_CONFIG_NO_IRQ
2100#define NRFX_WDT_CONFIG_NO_IRQ 0
2114#ifndef NRFX_WDT_DEFAULT_CONFIG_IRQ_PRIORITY
2115#define NRFX_WDT_DEFAULT_CONFIG_IRQ_PRIORITY 7
2120#ifndef NRFX_WDT_CONFIG_LOG_ENABLED
2121#define NRFX_WDT_CONFIG_LOG_ENABLED 0
2131#ifndef NRFX_WDT_CONFIG_LOG_LEVEL
2132#define NRFX_WDT_CONFIG_LOG_LEVEL 3
2147#ifndef NRFX_WDT_CONFIG_INFO_COLOR
2148#define NRFX_WDT_CONFIG_INFO_COLOR 0
2163#ifndef NRFX_WDT_CONFIG_DEBUG_COLOR
2164#define NRFX_WDT_CONFIG_DEBUG_COLOR 0