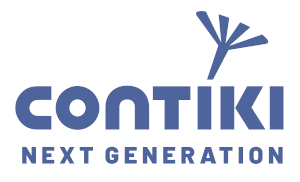 |
Contiki-NG
|
Loading...
Searching...
No Matches
20#ifndef __FLASH_LAYOUT_H__
21#define __FLASH_LAYOUT_H__
56#ifdef PSA_API_TEST_IPC
58#define FLASH_S_PARTITION_SIZE (0x48000)
59#define FLASH_NS_PARTITION_SIZE (0x28000)
61#define FLASH_S_PARTITION_SIZE (0x40000)
62#define FLASH_NS_PARTITION_SIZE (0x30000)
65#define FLASH_MAX_PARTITION_SIZE ((FLASH_S_PARTITION_SIZE > \
66 FLASH_NS_PARTITION_SIZE) ? \
67 FLASH_S_PARTITION_SIZE : \
68 FLASH_NS_PARTITION_SIZE)
71#define FLASH_AREA_IMAGE_SECTOR_SIZE (0x1000)
74#define FLASH_TOTAL_SIZE (0x100000)
77#define FLASH_BASE_ADDRESS (0x00000000)
85#define FLASH_AREA_BL2_OFFSET (0x0)
86#define FLASH_AREA_BL2_SIZE (0x10000)
88#if !defined(MCUBOOT_IMAGE_NUMBER) || (MCUBOOT_IMAGE_NUMBER == 1)
90#define FLASH_AREA_0_ID (1)
91#define FLASH_AREA_0_OFFSET (FLASH_AREA_BL2_OFFSET + FLASH_AREA_BL2_SIZE)
92#define FLASH_AREA_0_SIZE (FLASH_S_PARTITION_SIZE + \
93 FLASH_NS_PARTITION_SIZE)
95#define FLASH_AREA_2_ID (FLASH_AREA_0_ID + 1)
96#define FLASH_AREA_2_OFFSET (FLASH_AREA_0_OFFSET + FLASH_AREA_0_SIZE)
97#define FLASH_AREA_2_SIZE (FLASH_S_PARTITION_SIZE + \
98 FLASH_NS_PARTITION_SIZE)
102#define FLASH_AREA_SCRATCH_ID (FLASH_AREA_2_ID + 1)
103#define FLASH_AREA_SCRATCH_OFFSET (FLASH_AREA_2_OFFSET + FLASH_AREA_2_SIZE)
104#define FLASH_AREA_SCRATCH_SIZE (0)
106#define MCUBOOT_MAX_IMG_SECTORS ((FLASH_S_PARTITION_SIZE + \
107 FLASH_NS_PARTITION_SIZE) / \
108 FLASH_AREA_IMAGE_SECTOR_SIZE)
109#elif (MCUBOOT_IMAGE_NUMBER == 2)
111#define FLASH_AREA_0_ID (1)
112#define FLASH_AREA_0_OFFSET (FLASH_AREA_BL2_OFFSET + FLASH_AREA_BL2_SIZE)
113#define FLASH_AREA_0_SIZE (FLASH_S_PARTITION_SIZE)
115#define FLASH_AREA_1_ID (FLASH_AREA_0_ID + 1)
116#define FLASH_AREA_1_OFFSET (FLASH_AREA_0_OFFSET + FLASH_AREA_0_SIZE)
117#define FLASH_AREA_1_SIZE (FLASH_NS_PARTITION_SIZE)
119#define FLASH_AREA_2_ID (FLASH_AREA_1_ID + 1)
120#define FLASH_AREA_2_OFFSET (FLASH_AREA_1_OFFSET + FLASH_AREA_1_SIZE)
121#define FLASH_AREA_2_SIZE (FLASH_S_PARTITION_SIZE)
123#define FLASH_AREA_3_ID (FLASH_AREA_2_ID + 1)
124#define FLASH_AREA_3_OFFSET (FLASH_AREA_2_OFFSET + FLASH_AREA_2_SIZE)
125#define FLASH_AREA_3_SIZE (FLASH_NS_PARTITION_SIZE)
129#define FLASH_AREA_SCRATCH_ID (FLASH_AREA_3_ID + 1)
130#define FLASH_AREA_SCRATCH_OFFSET (FLASH_AREA_3_OFFSET + FLASH_AREA_3_SIZE)
131#define FLASH_AREA_SCRATCH_SIZE (0)
133#define MCUBOOT_MAX_IMG_SECTORS (FLASH_MAX_PARTITION_SIZE / \
134 FLASH_AREA_IMAGE_SECTOR_SIZE)
136#error "Only MCUBOOT_IMAGE_NUMBER 1 and 2 are supported!"
143#define MCUBOOT_STATUS_MAX_ENTRIES (0)
146#define FLASH_PS_AREA_OFFSET (FLASH_AREA_SCRATCH_OFFSET + \
147 FLASH_AREA_SCRATCH_SIZE)
148#define FLASH_PS_AREA_SIZE (0x4000)
151#define FLASH_ITS_AREA_OFFSET (FLASH_PS_AREA_OFFSET + \
153#define FLASH_ITS_AREA_SIZE (0x2000)
156#define FLASH_NV_COUNTERS_AREA_OFFSET (FLASH_ITS_AREA_OFFSET + \
158#define FLASH_NV_COUNTERS_AREA_SIZE (FLASH_AREA_IMAGE_SECTOR_SIZE)
161#define FLASH_MMIO_AREA_OFFSET (FLASH_NV_COUNTERS_AREA_OFFSET + \
162 FLASH_NV_COUNTERS_AREA_SIZE)
163#define FLASH_MMIO_AREA_SIZE (FLASH_AREA_IMAGE_SECTOR_SIZE)
166#define SECURE_IMAGE_OFFSET (0x0)
167#define SECURE_IMAGE_MAX_SIZE FLASH_S_PARTITION_SIZE
169#define NON_SECURE_IMAGE_OFFSET (SECURE_IMAGE_OFFSET + \
170 SECURE_IMAGE_MAX_SIZE)
171#define NON_SECURE_IMAGE_MAX_SIZE FLASH_NS_PARTITION_SIZE
176#define FLASH_DEV_NAME Driver_FLASH0
177#define TFM_HAL_FLASH_PROGRAM_UNIT (0x4)
183#define TFM_HAL_PS_FLASH_DRIVER Driver_FLASH0
189#define TFM_HAL_PS_FLASH_AREA_ADDR FLASH_PS_AREA_OFFSET
191#define TFM_HAL_PS_FLASH_AREA_SIZE FLASH_PS_AREA_SIZE
192#define PS_RAM_FS_SIZE TFM_HAL_PS_FLASH_AREA_SIZE
194#define TFM_HAL_PS_SECTORS_PER_BLOCK (1)
196#define TFM_HAL_PS_PROGRAM_UNIT (0x4)
204#define TFM_HAL_ITS_FLASH_DRIVER Driver_FLASH0
210#define TFM_HAL_ITS_FLASH_AREA_ADDR FLASH_ITS_AREA_OFFSET
212#define TFM_HAL_ITS_FLASH_AREA_SIZE FLASH_ITS_AREA_SIZE
213#define ITS_RAM_FS_SIZE TFM_HAL_ITS_FLASH_AREA_SIZE
215#define TFM_HAL_ITS_SECTORS_PER_BLOCK (1)
217#define TFM_HAL_ITS_PROGRAM_UNIT (0x4)
220#define TFM_NV_COUNTERS_AREA_ADDR FLASH_NV_COUNTERS_AREA_OFFSET
221#define TFM_NV_COUNTERS_AREA_SIZE (0x18)
222#define TFM_NV_COUNTERS_SECTOR_ADDR FLASH_NV_COUNTERS_AREA_OFFSET
223#define TFM_NV_COUNTERS_SECTOR_SIZE FLASH_AREA_IMAGE_SECTOR_SIZE
226#define FLASH_BASE_ADDRESS (0x00000000)
227#define S_ROM_ALIAS_BASE FLASH_BASE_ADDRESS
228#define NS_ROM_ALIAS_BASE FLASH_BASE_ADDRESS
231#define SRAM_BASE_ADDRESS (0x20000000)
232#define S_RAM_ALIAS_BASE SRAM_BASE_ADDRESS
233#define NS_RAM_ALIAS_BASE SRAM_BASE_ADDRESS
235#define TOTAL_ROM_SIZE FLASH_TOTAL_SIZE
236#define TOTAL_RAM_SIZE (0x00080000)